Assignment 3
Implementing a Class Hierarchy using C#
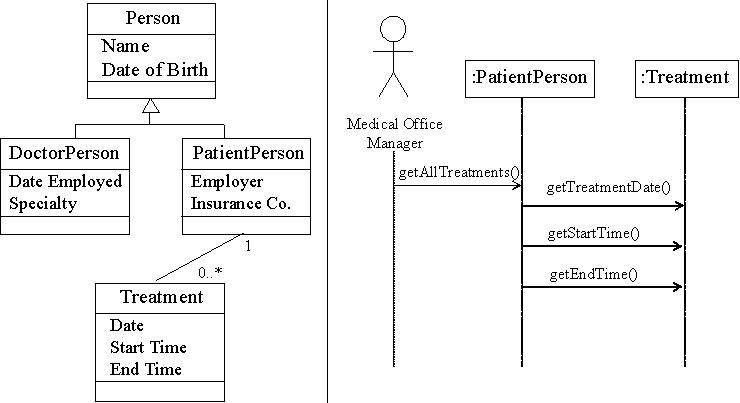
Translate the design structure to code in C#.
Assume appropriate attributes and services if required.
Person.cs
using System;
namespace AbstractFactory {
class Person {
private string
Name;
private Date DOB;
public
Person(string aName, Date aDOB) {
Name=aName;
Date=aDOB;
}
public void
setName(string aName) {Name=aName;}
public void
setDOB(Date aDOB) {DOB=aDOB;}
public string
getName() {return Name;}
public
Date getDOB() {return DOB;}
}
}
DoctorPerson.cs
using System;
namespace AbstractFactory {
class DoctorPerson : Person {
private
Date DateEmployed;
private string
Specialty;
public
DoctorPerson(Date aDate, string sSpec) {
DateEmployed=aDate;
Specialty=aSpec;
}
public void
setDateEmployed(Date aDate) {DateEmployed=aDate;}
public void
setSpecialty(string aSpec) {Specialty=aSpec;}
public
Date getDateEmployed() {return DateEmployed;}
public string
getSpecialty() {return Specialty;}
}
}
PatientPerson.cs
using System;
namespace AbstractFactory {
class PatientPerson : Person {
private string Employer;
private string Insurance;
private Treatment[] TreatArray;
private int NumOfTreatments;
public PatientPerson(string anEmployer,
string anInsurance) {
Employer=anEmployer;
Insurance=anInsurance;
NumOfTreatments=0;
}
public void setEmployer(string anEmployer) {Employer=anEmployer;}
public void setInsurance(string
anInsurance) {Insurance=anInsurance;}
public string getEmployer() {return
Employer;}
public string getInsurance() {return
Insurance;}
public int
getNumOfTreatments() {return NumOfTreatments;}
public void setTreatment(Date
aTreatDate, Time aStartTime, Time anEndTime) {
TreatArray[NumOfTreatments++]=new Treatment(aTreatDate, aStartTime, anEndTime);
}
public Treatment getTreatment(int
TreatNo) {return TreatArray[TreatNo];}
}
}
Treatment.cs
using System;
namespace AbstractFactory {
class Treatment {
private
Date TreatDate;
private
Time StartTime;
private
Time EndTime;
public
Treatment(Date aTreatDate, Time aStartTime, Time anEndTime) {
TreatDate=aTreatDate;
StartTime=aStartTime;
EndTime=anEndTime;
}
public void
setTreatmentDate(Date aTreatDate) {TreatDate=aTreatDate;}
public void
setStartTime(Time aStartTime) {StartTime=aStartTime;}
public void
setEndTime(Time anEndTime) {EndTime=anEndTime;}
public
Date getTreatmentDate() {return TreatDate;}
public
Time getStartTime() {return StartTime;}
public
Time getEndTime() {return EndTime;}
}
}
Done by: Low Hui Ting, Ivy (S9)
Back